Chapter 16. Classes and Functions
Code examples from this chapter are available from http://thinkpython.com/code/Time1.py.
Time
As another example of a user-defined type, we’ll define a class
called Time
that records the time of
day. The class definition looks like this:
class
Time
(
object
):
"""Represents the time of day.
attributes: hour, minute, second
"""
We can create a new Time
object
and assign attributes for hours, minutes, and seconds:
time
=
Time
()
time
.
hour
=
11
time
.
minute
=
59
time
.
second
=
30
The state diagram for the Time
object looks like Figure 16-1.
Exercise 16-1.
Write a function called print_time
that takes a Time object and prints
it in the form hour:minute:second
.
Hint: the format sequence '%.2d'
prints an integer using at least two
digits, including a leading zero if necessary.
Exercise 16-2.
Write a boolean function called is_after
that takes two Time objects, t1
and t2
, and returns True
if t1
follows t2
chronologically and False
otherwise. Challenge: don’t use an
if
statement.
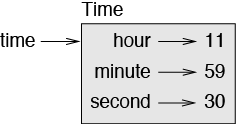
Figure 16-1. Object diagram.
Pure Functions
In the next few sections, we’ll write two functions that add time values. They demonstrate two kinds of functions: pure functions and modifiers. They also demonstrate a development plan I’ll call prototype and patch, which is a way of tackling a complex problem by starting with a simple prototype and incrementally dealing with the complications.
Here is ...
Get Think Python now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.