The Asynchronous Client/Server Pattern
In the ROUTER to DEALER example, we saw a 1-to-N use case where one server talks asynchronously to multiple workers. We can turn this upside down to get a very useful N-to-1 architecture where various clients talk to a single server, and do this asynchronously (Figure 3-12).
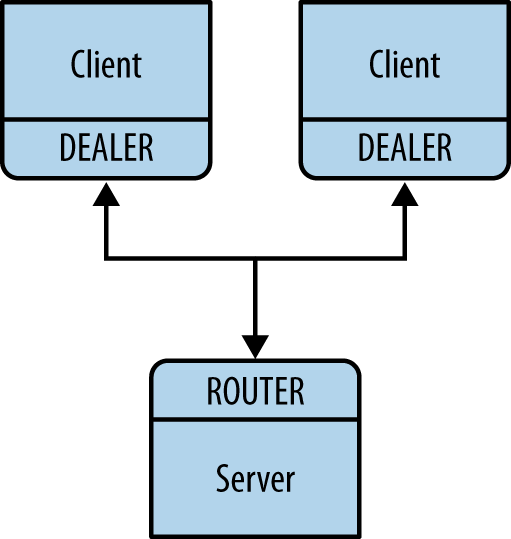
Figure 3-12. Asynchronous client/server
Hereâs how it works:
Clients connect to the server and send requests.
For each request, the server sends 0 or more replies.
Clients can send multiple requests without waiting for a reply.
Servers can send multiple replies without waiting for new requests.
Example 3-16 shows how this works.
Example 3-16. Asynchronous client/server (asyncsrv.c)
//
// Asynchronous client-to-server (DEALER to ROUTER)
//
// While this example runs in a single process, that is only to make
// it easier to start and stop the example. Each task has its own
// context and conceptually acts as a separate process.
#include "czmq.h"
// ---------------------------------------------------------------------
// This is our client task.
// It connects to the server, and then sends a request once per second.
// It collects responses as they arrive, and it prints them out. We will
// run several client tasks in parallel, each with a different random ID.
static
void
*
client_task
(
void
*
args
)
{
zctx_t
*
ctx
=
zctx_new
();
void
*
client
=
zsocket_new
(
ctx
,
ZMQ_DEALER
);
// Set ...
Get ZeroMQ now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.